Inheritance - Object-oriented programming (Parent-Child)
https://www.facebook.com/techaddaa/
Hey Friends,
This is third post in core java concepts blog. This series is specially designed for beginners to help them in their learning.
Introduction -
There are many concepts like Classes, Object, Polymorphism, Inheritance etc. As concluded in second post, here we will explore important concept called "inheritance" and learn types of inheritance, it's advantages along with implementation in java language.
This concept is simple to understand. Like in real world, every child get few habits from their parent inherently similarly in programming child classes inherit behaviour from parent class.
Parent class is also called super class, base class and child class is also called sub class, derived class.
This relationship is called as parent-child relationship or IS-A relationship. For example animal class in below diagram. Dog IS-A animal similarly Cat IS-A animal too. This is general guideline while implementing this, whenever you find such relationship between objects then we should use inheritance.
Prerequisite -
What is Inheritance?
The idea of inheritance is simple but powerful. When you want to create a new class and there is already a class that includes some of the code that you want, you can derive your new class from the existing class. While doing this, you can reuse the fields and methods of the existing class without having to write (and debug) them yourself.
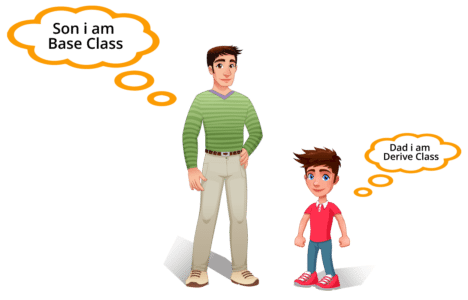
Types of inheritance -
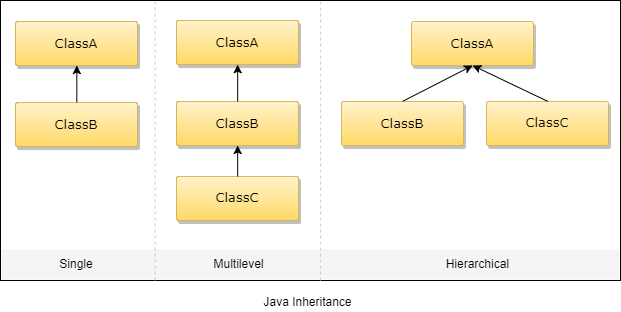
Implementation -
package com.utility;
public class Calc {
public void add(int a, int b) { // this method will add two numbers
System.out.println("a+b = " + a+b );
}
public void add(int a, int b, int c) { // this method will add three numbers
System.out.println("a+b+c = " + a+b+c );
}
public void add(String a, String b) { // this method will add two strings
System.out.println("a +b = " + a.concat(b) );
}
}
package com.utility;
public class AdvanceCalc extends Calc{
public void subtract(int a, int b) { // this method will subtract second number from first number
System.out.println("a-b = " + (a-b) );
}
}
package com.demo;
import com.utility.AdvanceCalc;
public class InheritanceDemo {
public static void main(String[] args) {
AdvanceCalc calc = new AdvanceCalc();
calc.add(5,6);
calc.subtract(50,10);
calc.add("Single"," Inheritance");
}
}
a+b = 56
a-b = 40
a +b = Single Inheritance
Process finished with exit code 0
Source code download -
https://github.com/sarveshoza/PolymorphismInJava/tree/feature/inheritance
Bonus Points -
- Default superclass: Except Object class, which has no superclass, every class has one and only one direct superclass (single inheritance). In the absence of any other explicit superclass, every class is implicitly a subclass of Object class.
- Superclass can only be one: A superclass can have any number of subclasses. But a subclass can have only one superclass. This is because Java does not support multiple inheritance with classes. Although with interfaces, multiple inheritance is supported by java.
- Inheriting Constructors: A subclass inherits all the members (fields, methods, and nested classes) from its superclass. Constructors are not members, so they are not inherited by subclasses, but the constructor of the superclass can be invoked from the subclass.
- Private member inheritance: A subclass does not inherit the private members of its parent class. However, if the superclass has public or protected methods(like getters and setters) for accessing its private fields, these can also be used by the subclass.
In sub-classes we can inherit members as is, replace them, hide them or supplement them with new members:
- The inherited fields can be used directly, just like any other fields.
- We can declare new fields in the subclass that are not in the superclass.
- The inherited methods can be used directly as they are.
- We can write a new instance method in the subclass that has the same signature as the one in the superclass, thus overriding it (as in example above, toString() method is overridden).
- We can write a new static method in the subclass that has the same signature as the one in the superclass, thus hiding it.
- We can declare new methods in the subclass that are not in the superclass.
- We can write a subclass constructor that invokes the constructor of the superclass, either implicitly or by using the keyword super.
- Don't get confuse between interface and inheritance.
1. Create class
2. Create Object
3. Define methods
4. Overload methods
5. Method Calling
6. Single Inheritance
Upcoming Posts -
1. Runtime Polymorphism - Part 2
2. Type of Inheritance - Part 2
3. Constructors in class
Comments
Post a Comment